
Thursday 14 May 2015
Operators in Java
Operators are symbols to use specify certain operation in the programming.
List of available operators,

Data types
Variables are nothing but reserved memory locations to store values. This means that when you create a variable you reserve some space in memory.
Based on the data type of a variable, the operating system allocates memory and decides what can be stored in the reserved memory. Therefore, by assigning different data types to variables, you can store integers, decimals, or characters in these variables.
There are two data types available in Java:
- Primitive Data Types
- Reference/Object Data Types
Variables
Variables
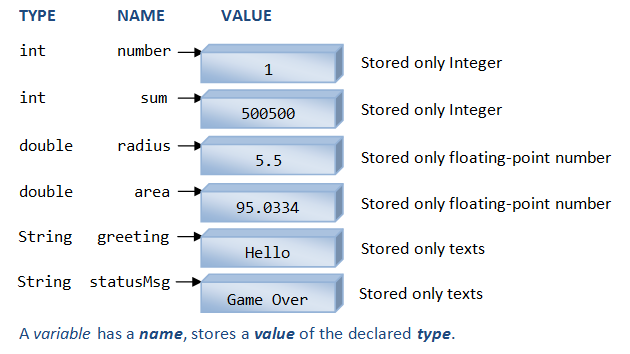
Variable is an place holder for data.We have 3 variables types in java.
- Local variables
- Instance variables
- Class/static variables
Local variables:
- Local variables are declared in methods, constructors, or blocks.
- Local variables are created when the method, constructor or block is entered and the variable will be destroyed once it exits the method, constructor or block.
- Access modifiers cannot be used for local variables.
- Local variables are visible only within the declared method, constructor or block.
- Local variables are implemented at stack level internally.
- There is no default value for local variables so local variables should be declared and an initial value should be assigned before the first use.
Example:
Here, age is a local variable. This is defined inside pupAge() method and its scope is limited to this method only.
public class Test{
public void pupAge(){
int age = 0;
age = age + 7;
System.out.println("Puppy age is : " + age);
}
public static void main(String args[]){
Test test = new Test();
test.pupAge();
}
}
This would produce the following result:
Puppy age is: 7
Example:
Following example uses age without initializing it, so it would give an error at the time of compilation.
public class Test {
public void pupAge(){
int age;
age = age + 7;
System.out.println("Puppy age is : " + age);
}
public static void main(String args[]){
Test test = new Test();
test.pupAge();
}
}
This would produce the following error while compiling it:
Test.java:4:variable number might not have been initialized age = age + 7; ^ 1 error
Instance variables:
- Instance variables are declared in a class, but outside a method, constructor or any block.
- When a space is allocated for an object in the heap, a slot for each instance variable value is created.
- Instance variables are created when an object is created with the use of the keyword 'new' and destroyed when the object is destroyed.
- Instance variables hold values that must be referenced by more than one method, constructor or block, or essential parts of an object's state that must be present throughout the class.
- Instance variables can be declared in class level before or after use.
- Access modifiers can be given for instance variables.
- The instance variables are visible for all methods, constructors and block in the class. Normally, it is recommended to make these variables private (access level). However visibility for subclasses can be given for these variables with the use of access modifiers.
- Instance variables have default values. For numbers the default value is 0, for Booleans it is false and for object references it is null. Values can be assigned during the declaration or within the constructor.
- Instance variables can be accessed directly by calling the variable name inside the class. However within static methods and different class ( when instance variables are given accessibility) should be called using the fully qualified name .ObjectReference.VariableName.
Example:
public class Employee{
// salary variable is a private static variable
private static double salary;
// DEPARTMENT is a constant
public static final String DEPARTMENT = "Development ";
public static void main(String args[]){
salary = 1000;
System.out.println(DEPARTMENT+"average salary:"+salary);
}
}
This would produce the following result:
name : Ransika
salary :1000.0
Class/static variables:
- Class variables also known as static variables are declared with the static keyword in a class, but outside a method, constructor or a block.
- There would only be one copy of each class variable per class, regardless of how many objects are created from it.
- Static variables are rarely used other than being declared as constants. Constants are variables that are declared as public/private, final and static. Constant variables never change from their initial value.
- Static variables are stored in static memory. It is rare to use static variables other than declared final and used as either public or private constants.
- Static variables are created when the program starts and destroyed when the program stops.
- Visibility is similar to instance variables. However, most static variables are declared public since they must be available for users of the class.
- Default values are same as instance variables. For numbers, the default value is 0; for Booleans, it is false; and for object references, it is null. Values can be assigned during the declaration or within the constructor. Additionally values can be assigned in special static initializer blocks.
- Static variables can be accessed by calling with the class name .ClassName.VariableName.
- When declaring class variables as public static final, then variables names (constants) are all in upper case. If the static variables are not public and final the naming syntax is the same as instance and local variables.
Example:
import java.io.*;
public class Employee{
// salary variable is a private static variable
private static double salary;
// DEPARTMENT is a constant
public static final String DEPARTMENT = "Development ";
public static void main(String args[]){
salary = 1000;
System.out.println(DEPARTMENT+"average salary:"+salary);
}
}
This would produce the following result:
Development average salary:1000
Note: If the variables are access from an outside class the constant should be accessed as Employee.DEPARTMENT
Packages
JDK packages structure
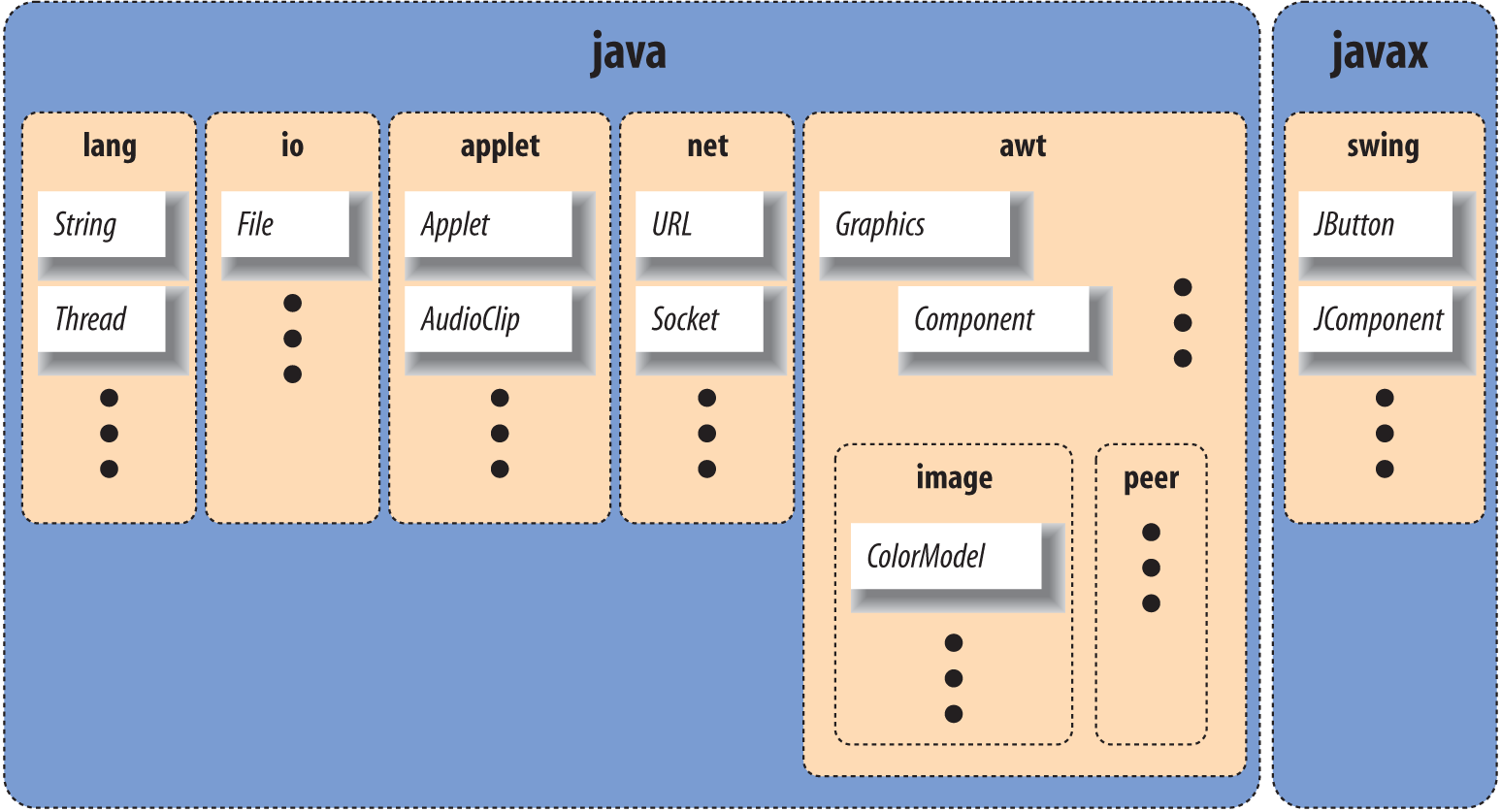
User defined package
User defined packages are nothing but placing the right class files in right place (package).
For example,
Consider a banking application which has multiple account opening procedures.
We will have an multiple account opening classes and placed under account opening package.
com.companyname.banking.accountopening
- SavingsAccountOpen
- FixedAccountOpen and etc..
Access modifiers
Access modifiers
Access modifiers are used in java programming to control the visibility of the data types,methods and classes.
Visibility reference,

Monday 11 May 2015
Features of Java
Features
Simple - Based upon C++ syntax,Avoided confusions like pointers,operator overloading and automatic garbage collection.
Object-Oriented - It's an simple methodology for software development and maintainence

Simple - Based upon C++ syntax,Avoided confusions like pointers,operator overloading and automatic garbage collection.
Object-Oriented - It's an simple methodology for software development and maintainence
- Object and class
Platform independent
Its an software based platform,contains run time environment (JRE) and (JDK) API
Its an software based platform,contains run time environment (JRE) and (JDK) API

Robust
- Robust simply means strong.
- Java uses strong memory management.
- Lack of pointers that avoids security problem.
- Automatic garbage collection in java.
- Exception handling and type checking mechanism in java.
Architecture neutral
- There is no implementation dependent features e.g. size of primitive types is set.

Portable
- We may carry the java byte code to any platform.

Dynamic
- Java is faster than traditional interpretation since byte code is "close" to native code still somewhat slower than a compiled language (e.g., C++).
Multi threaded
- A thread is like a separate program, executing concurrently.
- We can write Java programs that deal with many tasks at once by defining multiple threads.
- Main advantage of multi-threading is that it shares the same memory.
- Threads are important for multi-media, Web applications etc

Distributed
- RMI and EJB
Sunday 10 May 2015
Java Wiki
Java
- Is an programming language and a platform
- High level, robust, secured and object-oriented programming language
- Types - Standalone,Web,Enterprise and Mobile applications
- James Gosling, Mike Sheridan, and Patrick Naughton initiated the Java language project in June 1991. The small team of sun engineers called Green Team.
- Originally designed for small, embedded systems in electronic appliances like set-top boxes.
- Firstly, it was called "Green talk" by James Gosling and file extension was .gt
- After that, it was called Oak and was developed as a part of the Green project.
- Why Oak? Oak is a symbol of strength and choosen as a national tree of many countries like U.S.A., France, Germany, Romania etc.6) In 1995, Oak was renamed as "Java" because it was already a trademark by Oak Technologies
- Why they chooses java name for java language? The team gathered to choose a new name. The suggested words were "dynamic", "revolutionary", "Silk", "jolt", "DNA" etc. They wanted something that reflected the essence of the technology: revolutionary, dynamic, lively, cool, unique, and easy to spell and fun to say.
- According to James Gosling "Java was one of the top choices along with Silk". Since java was so unique, most of the team members preferred java.
- Java is an island of Indonesia where first coffee was produced (called java coffee).Notice that Java is just a name not an acronym.Originally developed by James Gosling at Sun Micro systems (which is now a subsidiary of Oracle Corporation) and released in 1995.In 1995, Time magazine called Java one of the Ten Best Products of 1995.
- JDK 1.0 released in(January 23, 1996).
Thursday 7 May 2015
Generics
Generics,

Introduction
- The Java Generics features were added to the Java language from Java 5.
- Generics add a way to specify concrete types to general purpose classes and methods that operated on
Object
before. - It sounds a bit abstract, so we will look at some examples using collections right away. Note: Generics can be used with other classes than the collection classes, but it is easiest to show the basics using collections.
The
List
interface represents a list of Object
instances. This means that we could put any object into a List
. Here is an example:List list = new ArrayList(); list.add(new Integer(2)); list.add("a String");
Because any object could be added, you would also have to cast any objects obtained from these objects. For instance:
Integer integer = (Integer) list.get(0); String string = (String) list.get(1);
Very often you only use a single type with a collection. For instance, you only keep
String
's or something else in the collection, and not mixed types like I did in the example above.
With Java's Generics features you can set the type of the collection to limit what kind of objects can be inserted into the collection. Additionally, you don't have to cast the values you obtain from the collection. Here is an example using Java's Generic's features:
List<String> strings = new ArrayList<String>(); strings.add("a String"); String aString = strings.get(0);
Nice, right?
Java 5 also got a new for-loop (also referred to as "for-each") which works well with generified collections. Here is an example:
List<String> strings = new ArrayList<String>(); //... add String instances to the strings list... for(String aString : strings){ System.out.println(aString); }
This for-each loop iterates through all
String
instances kept in the strings
list. For each iteration, the nextString
instance is assigned to the aString
variable. This for-loop is shorter than original while-loop where you would iterate the collections Iterator
and call Iterator.next()
to obtain the next instance.Vector
Vector,
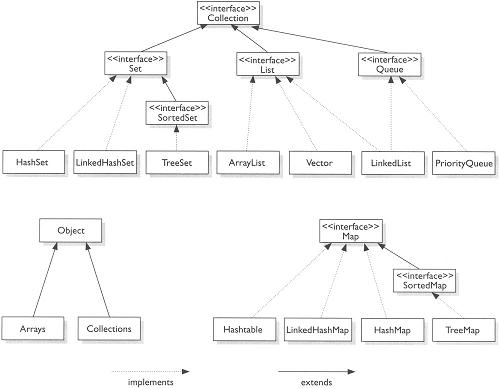
Vector implements a dynamic array. It is similar to ArrayList, but with two differences:
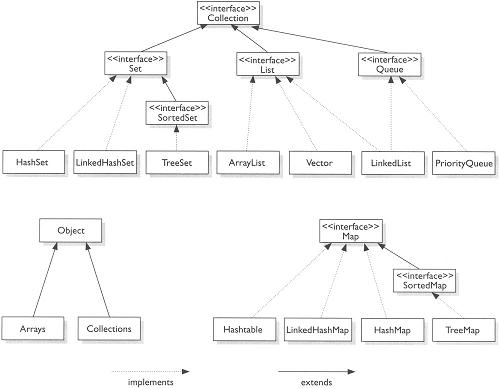
Vector implements a dynamic array. It is similar to ArrayList, but with two differences:
- Vector is synchronized.
- Vector contains many legacy methods that are not part of the collections framework.
- The Vector class implements a growable array of objects.
Like an array, it
contains components that can be accessed using an
integer index. However, the size of a Vector can grow or shrink as
needed to accommodate adding and removing items after the Vector has
been created.
Vector proves to be very useful if you don't know the size of the
array in advance or you just need one that can change sizes over the
lifetime of a program.
The Vector class supports four constructors. The first form creates a default vector, which has an initial size of 10:
Vector methods and its defintions,
https://docs.oracle.com/javase/7/docs/api/java/util/Vector.html
Here are the Vector constructors:
The first form creates a default vector, which has an initial size of 10.
The second form creates a vector whose initial capacity is specified by size.
The third form creates a vector whose initial capacity is specified by size and whose increment is specified by incr.
The increment specifies the number of elements to allocate each time that a vector is resized upward. The fourth form creates a vector that contains the elements of collection c. This constructor was added by Java 2.
The Vector class supports four constructors. The first form creates a default vector, which has an initial size of 10:
Vector methods and its defintions,
https://docs.oracle.com/javase/7/docs/api/java/util/Vector.html
Here are the Vector constructors:
Vector( )
Vector(int size)
Vector(int size, int incr)
Vector(Collection c)
The first form creates a default vector, which has an initial size of 10.
The second form creates a vector whose initial capacity is specified by size.
The third form creates a vector whose initial capacity is specified by size and whose increment is specified by incr.
The increment specifies the number of elements to allocate each time that a vector is resized upward. The fourth form creates a vector that contains the elements of collection c. This constructor was added by Java 2.
Example,
Get the vector capacity,
v.capacity();
// initial size is 3, increment is 2
Vector v = new Vector(3, 2);
v.addElement(new Integer(1));
Get the vector capacity,
v.capacity();
Producer and Consumer - Wait(),Notify
Producer and consumer example for wait(),notify() of thread
import java.util.Vector; class Producer extends Thread { static final int MAXQUEUE = 5; private Vector messages = new Vector(); @Override public void run() { try { while (true) { putMessage(); //sleep(5000); } } catch (InterruptedException e) { } } private synchronized void putMessage() throws InterruptedException { while (messages.size() == MAXQUEUE) { wait(); } messages.addElement(new java.util.Date().toString()); System.out.println("put message"); notify(); //Later, when the necessary event happens, the thread
that is running it calls notify() from a block synchronized on the same object. } // Called by Consumer public synchronized String getMessage() throws InterruptedException { notify(); while (messages.size() == 0) { wait();//By executing wait() from a synchronized block,
a thread gives up its hold on the lock and goes to sleep. } String message = (String) messages.firstElement(); messages.removeElement(message); return message; } } class Consumer extends Thread { Producer producer; Consumer(Producer p) { producer = p; } @Override public void run() { try { while (true) { String message = producer.getMessage(); System.out.println("Got message: " + message); //sleep(200); } } catch (InterruptedException e) { e.printStackTrace(); } } public static void main(String args[]) { Producer producer = new Producer(); producer.start(); new Consumer(producer).start(); } }
Output
Got message: Fri Mar 02 21:37:21 EST 2015 put message put message put message put message put message Got message: Fri Mar 02 21:37:21 EST 2015
Got message: Fri Mar 02 21:37:21 EST 2015
Got message: Fri Mar 02 21:37:21 EST 2015
Got message: Fri Mar 02 21:37:21 EST 2015
Got message: Fri Mar 02 21:37:21 EST 2015 put message put message put message put message put message
Got message: Fri Mar 02 21:37:21 EST 2015
Got message: Fri Mar 02 21:37:21 EST 2015
Got message: Fri Mar 02 21:37:21 EST 2015
Hash table
Hash table
- A Hashtable is an array of list.Each list is known as a bucket.The position of bucket is identified by calling the hashcode() method.A Hashtable contains values based on the key. It implements the Map interface and extends Dictionary class.
- It contains only unique elements.
- It may have not have any null key or value.
- It is synchronized.
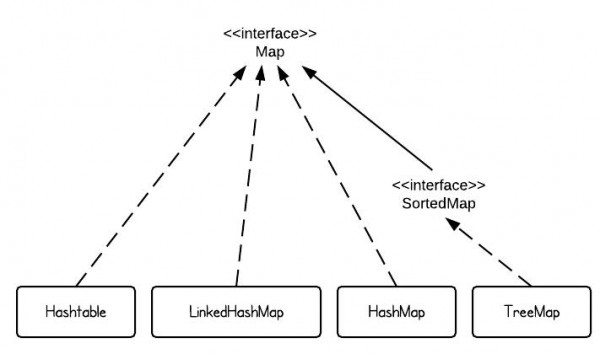
In computing, a hash table (hash map) is a data structure used to implement an associative array, a structure that can map keys to values. A hash table uses a hash function to compute an index into an array of buckets or slots, from which the correct value can be found.
N | Methods with Description |
---|---|
1 | void clear( )
Resets and empties the hash table.
|
2 | Object clone( )
Returns a duplicate of the invoking object.
|
3 | boolean contains(Object value)
Returns true if some value equal to value exists within the hash table. Returns false if the value isn't found.
|
4 | boolean containsKey(Object key)
Returns true if some key equal to key exists within the hash table. Returns false if the key isn't found.
|
5 | boolean containsValue(Object value)
Returns true if some value equal to value exists within the hash table. Returns false if the value isn't found.
|
6 | Enumeration elements( )
Returns an enumeration of the values contained in the hash table.
|
7 | Object get(Object key)
Returns the object that contains the value associated with key. If key is not in the hash table, a null object is returned.
|
8 | boolean isEmpty( )
Returns true if the hash table is empty; returns false if it contains at least one key.
|
9 | Enumeration keys( )
Returns an enumeration of the keys contained in the hash table.
|
10 | Object put(Object key, Object value)
Inserts a key and a value into the hash table. Returns null if key isn't already in the hash table; returns the previous value associated with key if key is already in the hash table.
|
11 | void rehash( )
Increases the size of the hash table and rehashes all of its keys.
|
12 | Object remove(Object key)
Removes key and its value. Returns the value associated with key. If key is not in the hash table, a null object is returned.
|
13 | int size( )
Returns the number of entries in the hash table.
|
14 | String toString( )
Returns the string equivalent of a hash
|
Example,
Hashtable<Integer,String> table = new Hashtable<Integer,String>();
table.put(1,"what's app");
table.put(2,"skype");
for(Map.Entry m:hm.entrySet()){
System.out.println(m.getKey()+" "+m.getValue());
}
Output :
1 what's app
2 skype
HashMap and Hashtable both are used to store data in key and value form. Both are using hashing technique to store unique keys.
But there are many differences between HashMap and Hashtable classes that are given below.
HashMap
|
Hashtable
|
1) HashMap is non
synchronized. It is not-thread safe and can't
be shared between many threads without proper synchronization code.
|
Hashtable is synchronized. It is thread-safe and can be shared with many threads.
|
2) HashMap allows
one null key and multiple null values.
|
Hashtable doesn't
allow any null key or value.
|
3) HashMap is a new
class introduced in JDK 1.2.
|
Hashtable is a legacy
class.
|
4) HashMap is fast.
|
Hashtable is slow.
|
5) We can make the HashMap as
synchronized by calling this code
Map m =
Collections.synchronizedMap(hashMap);
|
Hashtable is internally
synchronized and can't be unsynchronized.
|
6) HashMap is traversed
by Iterator.
|
Hashtable is traversed
by Enumerator and Iterator.
|
7) Iterator in HashMap is fail-fast.
|
Enumerator in Hashtable is not
fail-fast.
|
8) HashMap inherits AbstractMap class.
|
Hashtable inherits Dictionary class.
|
Subscribe to:
Posts (Atom)