Normally, array is a collection of similar type of elements that have contiguous memory location.
Java array is an object the contains elements of similar data type. It is a data structure where we store similar elements. We can store only fixed set of elements in a java array.
Array in java is index based, first element of the array is stored at 0 index.
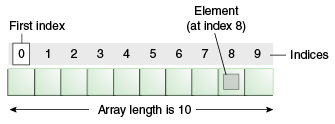
Types of array,
Single dimensional array
Multi dimensional array
Single dimensional array
Syntax,
dataType[] arr; (or) dataType []arr; (or) dataType arr[];
Instantiation of an Array,
arrayVariable =new datatype[size];
Sample
int a[]=new int[3];//declaration and instantiation
a[0]=10;//initialization
a[1]=20;
a[2]=70;
//printing array
for(int i=0;i<a.length;i++) { //length is the property of array
System.out.println(a[i]);
}
Output : 10,20,30
int a[]={33,3,4,5};//declaration, instantiation and initialization
//printing array
for(int i=0;i<a.length;i++)//length is the property of array {
System.out.println(a[i]);
}
Multi dimensional array
Syntax,
dataType[][] referenceVariable; (or)
dataType [][]referenceVariable; (or)
dataType referenceVariable[][]; (or)
dataType []referenceVariable[];
Instantiation
int[][] arr=new int[3][3];//3 row and 3 column
Initialize,
arr[0][0]=1;
//declaring and initializing 2D array
int arr[][]={{1,2,3},{2,4,5},{4,4,5}};
//printing 2D array
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}}
Output:1 2 3
2 4 5
4 4 5
a[1]=20;
a[2]=70;
//printing array
for(int i=0;i<a.length;i++) { //length is the property of array
System.out.println(a[i]);
}
Output : 10,20,30
int a[]={33,3,4,5};//declaration, instantiation and initialization
//printing array
for(int i=0;i<a.length;i++)//length is the property of array {
System.out.println(a[i]);
}
Multi dimensional array
Syntax,
dataType[][] referenceVariable; (or)
dataType [][]referenceVariable; (or)
dataType referenceVariable[][]; (or)
dataType []referenceVariable[];
Instantiation
int[][] arr=new int[3][3];//3 row and 3 column
Initialize,
arr[0][0]=1;
arr[0][1]=2;
arr[0][2]=3;
arr[1][0]=4;
arr[1][1]=5;
arr[1][2]=6;
arr[2][0]=7;
arr[2][1]=8;
arr[2][2]=9;
int arr[][]={{1,2,3},{2,4,5},{4,4,5}};
//printing 2D array
for(int i=0;i<3;i++){
for(int j=0;j<3;j++){
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}}
Output:1 2 3
2 4 5
4 4 5
Advantages
- An array know the type it holds, i.e., compile-time type checking.
- An array know its size, i.e., ask for the length.
- An array can hold primitive types directly.
- An array can only hold one type of objects (including primitives).
- Arrays are fixed size.
Helper class,
java.util.Arrays
java.util.Arrays
- Search and sort: binarySearch(), sort()
- Comparison: equals() (many overloaded)
- Instantiation: fill() (many overloaded)
- Conversion: asList()
No comments:
Post a Comment