Hash table
- A Hashtable is an array of list.Each list is known as a bucket.The position of bucket is identified by calling the hashcode() method.A Hashtable contains values based on the key. It implements the Map interface and extends Dictionary class.
- It contains only unique elements.
- It may have not have any null key or value.
- It is synchronized.
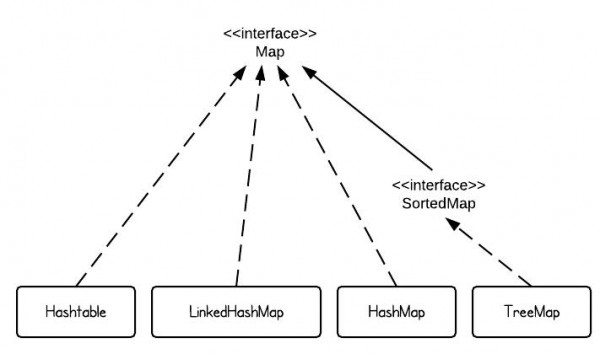
In computing, a hash table (hash map) is a data structure used to implement an associative array, a structure that can map keys to values. A hash table uses a hash function to compute an index into an array of buckets or slots, from which the correct value can be found.
N | Methods with Description |
---|---|
1 | void clear( )
Resets and empties the hash table.
|
2 | Object clone( )
Returns a duplicate of the invoking object.
|
3 | boolean contains(Object value)
Returns true if some value equal to value exists within the hash table. Returns false if the value isn't found.
|
4 | boolean containsKey(Object key)
Returns true if some key equal to key exists within the hash table. Returns false if the key isn't found.
|
5 | boolean containsValue(Object value)
Returns true if some value equal to value exists within the hash table. Returns false if the value isn't found.
|
6 | Enumeration elements( )
Returns an enumeration of the values contained in the hash table.
|
7 | Object get(Object key)
Returns the object that contains the value associated with key. If key is not in the hash table, a null object is returned.
|
8 | boolean isEmpty( )
Returns true if the hash table is empty; returns false if it contains at least one key.
|
9 | Enumeration keys( )
Returns an enumeration of the keys contained in the hash table.
|
10 | Object put(Object key, Object value)
Inserts a key and a value into the hash table. Returns null if key isn't already in the hash table; returns the previous value associated with key if key is already in the hash table.
|
11 | void rehash( )
Increases the size of the hash table and rehashes all of its keys.
|
12 | Object remove(Object key)
Removes key and its value. Returns the value associated with key. If key is not in the hash table, a null object is returned.
|
13 | int size( )
Returns the number of entries in the hash table.
|
14 | String toString( )
Returns the string equivalent of a hash
|
Example,
Hashtable<Integer,String> table = new Hashtable<Integer,String>();
table.put(1,"what's app");
table.put(2,"skype");
for(Map.Entry m:hm.entrySet()){
System.out.println(m.getKey()+" "+m.getValue());
}
Output :
1 what's app
2 skype
HashMap and Hashtable both are used to store data in key and value form. Both are using hashing technique to store unique keys.
But there are many differences between HashMap and Hashtable classes that are given below.
HashMap
|
Hashtable
|
1) HashMap is non
synchronized. It is not-thread safe and can't
be shared between many threads without proper synchronization code.
|
Hashtable is synchronized. It is thread-safe and can be shared with many threads.
|
2) HashMap allows
one null key and multiple null values.
|
Hashtable doesn't
allow any null key or value.
|
3) HashMap is a new
class introduced in JDK 1.2.
|
Hashtable is a legacy
class.
|
4) HashMap is fast.
|
Hashtable is slow.
|
5) We can make the HashMap as
synchronized by calling this code
Map m =
Collections.synchronizedMap(hashMap);
|
Hashtable is internally
synchronized and can't be unsynchronized.
|
6) HashMap is traversed
by Iterator.
|
Hashtable is traversed
by Enumerator and Iterator.
|
7) Iterator in HashMap is fail-fast.
|
Enumerator in Hashtable is not
fail-fast.
|
8) HashMap inherits AbstractMap class.
|
Hashtable inherits Dictionary class.
|
No comments:
Post a Comment